In today’s fast-paced mobile development landscape, ensuring optimal performance is crucial. React Native has become a go-to framework for building cross-platform applications, but like any technology, it can suffer from performance issues like memory leaks. This comprehensive guide will walk you through the causes of memory leaks in React Native, how to debug them using the latest techniques and tools, and provide practical code examples to help you resolve these issues.
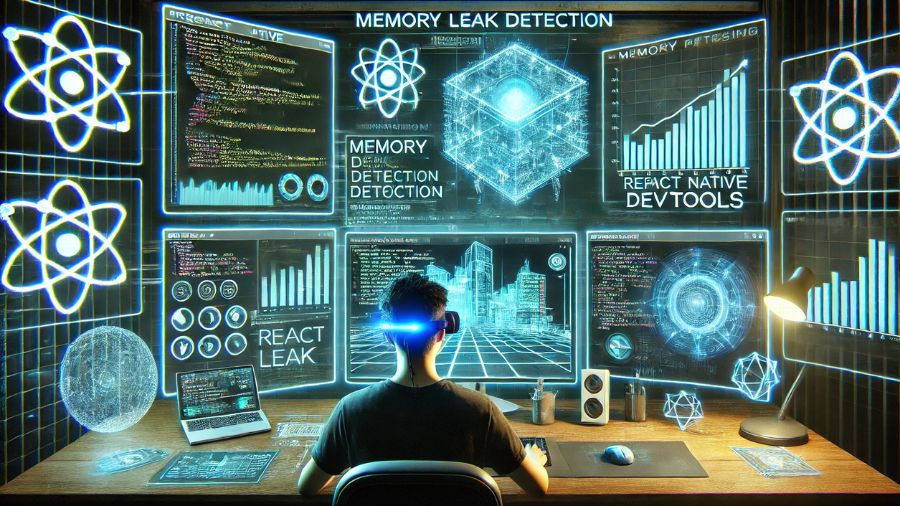
Table of Contents
- Introduction
- Understanding Memory Leaks in React Native
- Identification of memory leaks
- Fixing Memory Leaks in React Native
- Latest equipment for debugging memory leak
- Best practice to stop memory leaks
- Conclusion
1. Introduction
Memory leaks if found in React Native can very badly hamper the performance of any app, slow responsiveness, caused display glitches and high memory consumption, or can wait for hangs to crash. This entirely degrades user experience and makes the troubleshooting of its issues complex. Locating and eliminating the memory leak is important for having a smooth and stable application.
The guide will discuss new debugging techniques and tools that successfully help check for issues in memory leak detection and prevention. Best practices related to monitoring memory usage, understanding garbage collection, and effective performance optimization to avert leaks to begin with, will learn here.
Also, about memory leaks induced by inefficient event listeners, unmounted components, and improper asynchronous calls in addition, will come under this subheading. Proper cleanup functions and lifecycle management techniques can keep your application much more effective.
2. Understand the memory leaks in react native
A memory leak occurs when a program holds onto references to objects that are no longer deemed necessary for garbage collection. This eventually leads to memory bloat, slow performance, and unresponsive behavior. In React Native, memory leaks could adversely affect app stability and communication.
Common culprits include unmounted components still retaining resources, unchanged timers or event listeners, and closures capturing undesirable contexts. And poor data handling and caching decisions only run higher on memory.
Unchecked, these issues slow performance, cause UI lag, or even crash the app. Developers must properly implement the cleanup function, dispose of unwanted event listeners, and manage memory efficiently throughout the lifecycle. Fixing these will ensure an uninterrupted performance of the app and a smooth user experience with no unanticipated slowdowns or crashes.
3. Identification of memory leaks
Identifying and fixing memory leaks leaked in natives
Before fixing the memory leak, you first need to identify where it is happening. The memory leak occurs when an application fails to release memory which is no longer in use, causing unnecessary resource consumption.
See for performance fall
One of the initial signs of memory leak is a performance fall. If your app slows down considerably over time, often crashes due to out-of-mery (OOM) errors, or starts consuming excessive RAM, a memory leak may be the reason for this. Some common indicators of memory leaks include:
Increase in load time – It may take longer to start the app, recover data or submit components.
Unwanted – UI elements can be freeze or dull during interaction.
High CPU and GPU use – excessive memory consumption can pose additional stress on the processor, causing overheating and rapid battery drain.
Stuttering animations – If your app has animation or infection, they can be irritable or fail to run smoothly.
If the memory leak becomes uncontrolled, they can significantly affect the user experience. Over time, they can cause your app freeze, stuttering, or even unexpectedly closed, especially during intensive tasks. To prevent this, developers should actively monitor memory use and compare resource consumption in various sessions.
Use Performance Monitoring Equipment
To effectively identify the memory leaks, developers must take advantage of performing monitoring equipment that track the memory use over time. React native provides various debugging solutions, including flipper, excode instruments (for iOS),
4. Fixing Memory Leaks in React Native
Once you identify the memory leak, apply these techniques to solve them:
1. Current cleaning phenomenon listeners and timer
When using the event listeners, make sure that they are removed when the components are unmotted.
useEffect(() => {
const handleResize = () => console.log("Window resized");
window.addEventListener("resize", handleResize);
return () => {
window.removeEventListener("resize", handleResize);
};
}, []);
2. Avoid storing large items in the state
Large items kept in a state are notorious for causing memory issues resulting in performance and responsiveness problems of the application. Memory issues may arise with the storage of several types of data such as big JSON objects, huge lists, or media files; this instigates unnecessary re-renders, delays UI updates, and eats up memory. Instead, allow only crucial data to be stored and the state management to be optimized by inducing lazy loading, pagination, and offloading into external storage mechanisms like local databases or cloud storage. Use compact data structures and keep state to a minimum so as to help with performance and optimization of memory, thereby forming a smooth experience for application monetization.
3. Use weak references in closure
Getting unneeded contexts captured in closures causes them to be unreachable for garbage collection, which in turn gives rise to memory leaks. Strong references captured by closures stop the actual object from being freed, which results in increased memory consumption over time.
Using the WeakRef APIs in JavaScript, create weak references to those objects not needed to persist. By doing so, they get eligible for garbage collection whenever other strong references cease to exist. This process helps in clearing memory from event listeners, timers, or error data, hindering memory bloat and performance issues.
4. Components optimize life cycle methods
In the components of the square, always clean into the ingredient virtue.
componentWillUnmount() {
this.setState = () => null;
}
5. Use virtualized lists for large data sets
Using a flatlist
instead of scrollview
improves memory efficiency by presenting only visible items.
<FlatList
data={data}
renderItem={({ item }) => <Text>{item.name}</Text>}
keyExtractor={(item) => item.id.toString()}
/>
5. Latest equipment for debugging memory leak
Flipper
Flipper for React Native is a robust debugging utility that enables developers to measure and optimize application performance. It provides significant features like memory tracking, performance analysis, and plugin functionality. Flipper can be utilized by developers to detect memory leaks, track resource usage, and debug performance issues with ease. With Flipper integrated, developers can have real-time views of how their apps are behaving and debug and avoid unnecessary memory consumption that would cause the app to slow down or crash.
Flipper also has a range of plugins, which allow for greater support for profiling, network inspections, and layout debugging. That is what makes Flipper such a lifesaver when it comes to optimizing React Native apps. Whether one is dealing with memory leak tracing, performance bottleneck profiling, or UI bug debugging, Flipper creates a central platform whereby such operations are optimized. However, build errors developers may get when using Flipper to integrate with React Native, especially Android, are certain to occur.
import whyDidYouRender from '@welldone-software/why-did-you-render';
whyDidYouRender(React, {
trackAllPureComponents: true,
});
6. Best practice to stop memory leaks
1. Use functional components and hooks
The functional component and hook response provides a modern and efficient way to create applications. Unlike square components, which rely on life -cycle methods, allow hook developers to manage state and side effects within functional components. It improves results in cleaner, more readable code with low lines and re -formation. Hooks such as usestate and useeffect help to handle dynamic data and perseverance efficiently, reducing unnecessary render and enhancing performance. Taking advantage of the hook, developers can write modular and maintainable codes, making development easier for scalable applications. For a detailed guide on React hooks .
2. Reduce global state dependence
It increases the memory demand with excessive global state usage, complicates the maintenance, and apparently results in unexpected behaviors. View states become hard to track in huge applications. States are changed whenever parts of the application are trying to use them concurrently, thus complicating the debugging process. Such dependencies might weaken stability while reducing performance, especially on mobile devices. Repeated changes lead to unnecessary re-renders, which affects the UI by slowing it down. Hence, eliminating global state dependency will improve efficiency, scalability, and the performance of any application.
Use Performance Monitoring Equipment
To effectively identify memory leaks, developers should utilize performance monitoring tools that track memory usage over time. React Native provides various debugging solutions, including:
- Flipper – A debugging platform for inspecting React Native apps.
- Xcode Instruments (for iOS) – Helps track memory allocations and leaks.
Conclusion
Identifying and fixing memory leaks leaked in natives
Before fixing the memory leak, you first need to identify where it is happening. The memory leak occurs when an application fails to release memory which is no longer in use, causing unnecessary resource consumption. Over time, it can slow down the app, reduce performance, and cause crash. Here are some of the major signs and techniques that help you detect memory leaks in your react country application.
See for performance fall
One of the initial signs of memory leak is a performance fall. If your app slows down considerably over time, often crashes due to out-of-mery (OOM) errors, or starts consuming excessive RAM, a memory leak may be the reason for this. Some common indicators of memory leaks include:
Increase in load time
It may take longer to start the app, recover data or submit components.
Unwanted
UI elements can be freeze or dull during interaction.
High CPU and GPU use
Excessive memory consumption can pose additional stress on the processor, causing overheating and rapid battery drain.
Stuttering animations
If your app has animation or infection, they can be irritable or fail to run smoothly.
If you new to React Native start with this React Native Tutorial for Beginners to Construct a strong foundation. Reason effect concepts makes it easier to important and set store leaks inch respond indigenous apps. Memory leaks can slow down Effectiveness cause display glitches and increase memory consumption leading to crashes. These Problems negatively affect exploiter get and get debugging hard. Proper memory management and leak prevention are essential for ensuring a smooth high-performing React Native Use.